Introduction
Github Actions allow you to run builds and other functions related to your codebase, but sometimes other people need to be made aware of output from those workflows, along with details of any failures which may have arisen. In this guide, we'll create a Github action which fails immediately (intentionally), and add failure handling which interacts with a Slack webhook to notify channel members when a job fails to complete.
Assumptions
- A Github repository, with permission to create Actions and commit changes
- A Slack webhook URL, or permission to create one within a workspace
- Familiarity with YAML is beneficial, but not required
Steps
The simple option: Github Slack app
Github has a Slack app which can be added directly into your workspace, which includes commands to allow the creation of issues direct from your channel, as well as notifications for a variety of events, such as commits and pull requests.
The reason you're reading this guide is likely because you want to get those notifications, and the simplest solution to your problem is to just add the Github app to your workspace, and enter the following into your channel of choice:
/github subscribe accountname/repositoryname workflows:{name:"workflow-name"}
This will subscribe your channel to receive notifications for issues, pulls, commits, releases and deployments by default, along with your specified workflow.
If you only care about the workflow, you can unsubscribe from the others by entering:
/github unsubscribe accountname/repositoryname issues, pulls, commits, releases, deployments
You can add further filtering to this by refining the workflow subscription to a particular event
, branch
or actor
(user).
Limitations of the Slack app
This may be fine for your needs, but if all you want is notifications for failed workflows, you might find that your channel is being filled up with lots of useless information which causes the important stuff to get lost in the noise.
At the time of writing, there is an open issue on Github requesting the implementation of additional filtering, however this was raised over a year ago, and is yet to receive any response from the maintainer.
A further limitation of the Slack app is that it doesn't allow you to customise the channel output, for example, you may want to display a specific message, include a related URL, or tag certain channel members.
The alternative: app webhooks
Slack webhooks are the modern equivalent to the Incoming Webhooks app. The original app worked really well but will soon be deprecated, and is no longer recommended for use.
Create a Slack webhook
You'll first need to create a Slack app if you don't already have one. It's a simple process, and I've documented the process as part of another article, Send messages to Slack with a custom Slack Bot.
Once you have an app, navigate to the "Incoming Webhooks" page, and flick the toggle to "On".
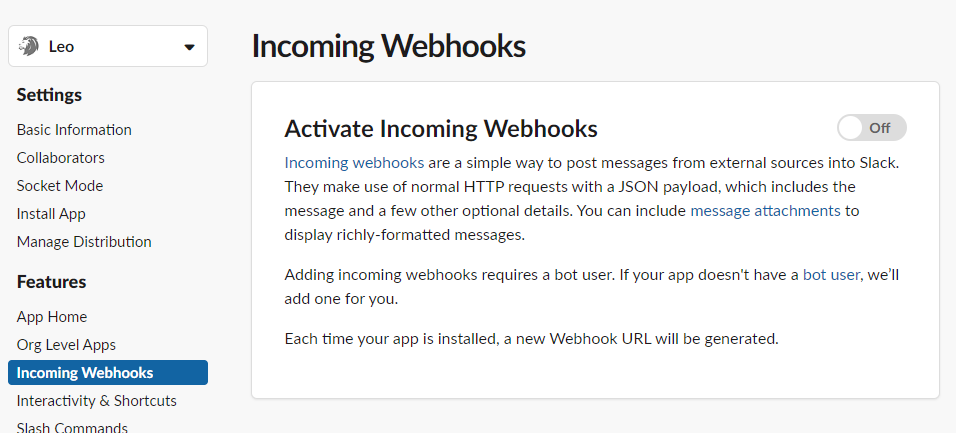
If you need to update the permissions for your app, a banner will appear along the top to authorise the change. Once approved, you'll be returned to the previous screen.
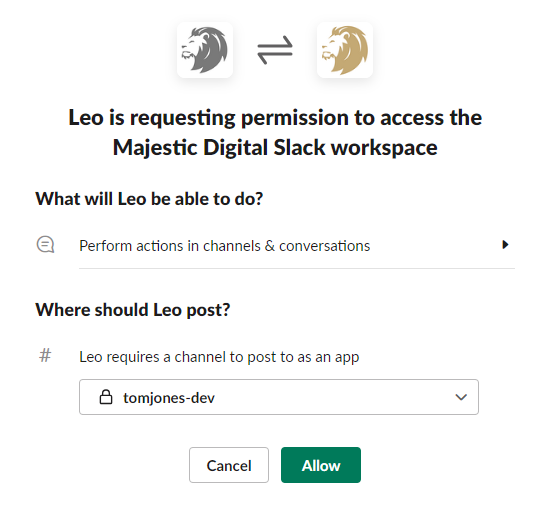
Test the integration and webhook URL
If you look at your Slack workspace, you should see your app has been added to the channel.

Back on the Settings page of your workspace, you'll see the webhook URL which can be copied. You can test the Slack app integration and URL are functioning properly before moving onto the next steps in Github by running the following command in PowerShell and ensuring the message is posted in your channel:
Invoke-RestMethod -Method POST -Body '{"text":"Hello, world!"}' https://hooks.slack.com/services/....your-webhook-url
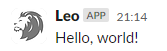
Add a secret to your Github repository
In your repository, go to Settings Security Secrets and variables Actions and click .
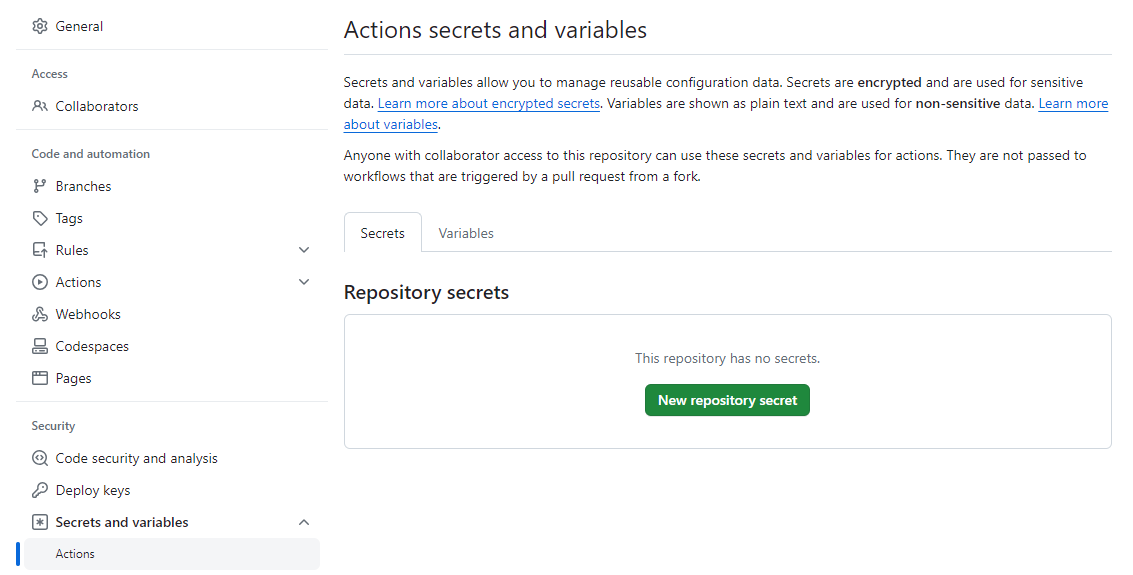
Give it a meaningful name, such as "SLACK_WEBHOOK_URL", and paste the URL you were provided earlier in the field below.
Add a failure job to your Github workflow
Navigate to the workflow which you need failure notifications for, and add the on-failure
job block shown in the below example to the end of your .yaml file, ensuing it's indented correctly to be contained within the jobs
block.
name: Failure Notification
on:
push:
branches: [ "tomjonesdev-patch-1" ]
jobs:
failing-job:
runs-on: ubuntu-latest
steps:
- name: failing step
run: exit 1
on-failure:
runs-on: ubuntu-latest
if: ${{ always() && (needs.failing-job.result == 'failure' || needs.failing-job.result == 'timed_out') }}
needs:
- failing-job
steps:
- uses: actions/checkout@v2
- name: Slack Notification
uses: rtCamp/action-slack-notify@v2
env:
SLACK_USERNAME: Leo
SLACK_TITLE: Workflow ${{ needs.failing-job.result }}
MSG_MINIMAL: actions url
SLACK_WEBHOOK: ${{ secrets.SLACK_WEBHOOK_URL }}
This example file creates a simple job (failing-job
) which fails immediately using an exit code, then the on-failure
job:
- makes use of the Slack Notify action (
uses
) - always runs, regardless of any other events in the workflow (
always()
) - only executes after the first job has ended (
needs
) - only executes if the first job fails or times out
- grabs the Slack webhook URL from the secret we created earlier (
SLACK_WEBHOOK
), then - generates a Slack message with the remaining parameters.
A full description of the options available can be found in the Slack Notify documentation.
Run your failing workflow
Run the workflow by pushing a commit to the branch specified in the on
block shown above. Ensure you've changed this value to your own branch name, or chosen a different Github Action trigger. Once the workflow is complete, you should see the first job failing immediately, and the on-failure
job completing successfully shortly after.
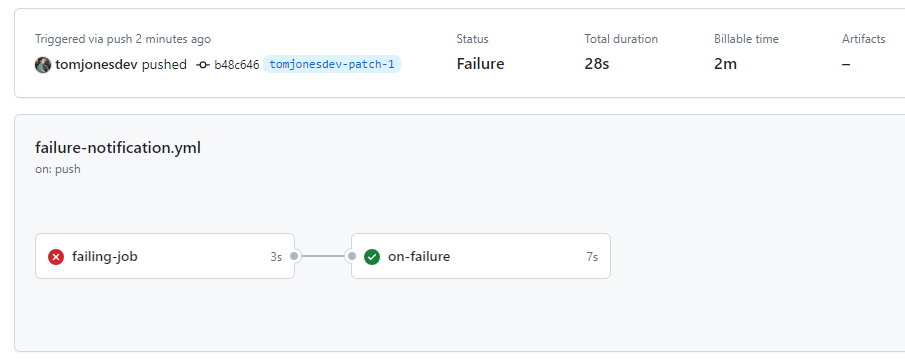
If everything looks good here, you should also have seen a notification pop up in your Slack channel.
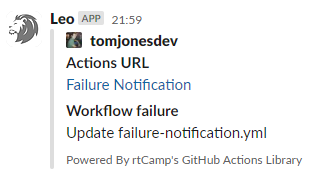
Once you're confident that failures are being properly notified, change the failing-job
references in the .yml file to the actual job you want to receive notifications for.